问题:
给你一个二叉树的根节点 root
,判断其是否是一个有效的二叉搜索树。
有效 二叉搜索树定义如下:
- 节点的左子树只包含 小于 当前节点的数。
- 节点的右子树只包含 大于 当前节点的数。
- 所有左子树和右子树自身必须也是二叉搜索树。
示例 1:
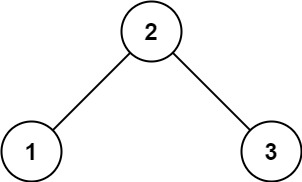
输入:root = [2,1,3] 输出:true
示例 2:
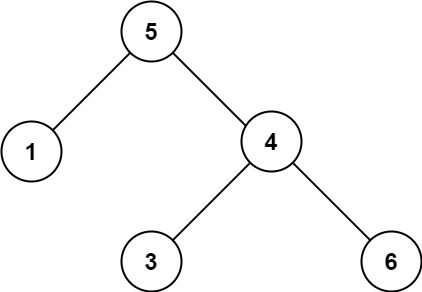
输入:root = [5,1,4,null,null,3,6] 输出:false 解释:根节点的值是 5 ,但是右子节点的值是 4 。
提示:
- 树中节点数目范围在
[1, 104]
内 -231 <= Node.val <= 231 - 1
Related Topics
题解:
// @lc code=start
/**
* Definition for a binary tree node.
* type TreeNode struct {
* Val int
* Left *TreeNode
* Right *TreeNode
* }
*/
func isValidBST(root *TreeNode) bool {
return isValid(root, nil, nil)
}
func isValid(root *TreeNode, min *int, max *int) bool {
if root.Left == nil && root.Right == nil {
return true
}
if root.Left != nil {
if root.Left.Val >= root.Val {
return false
}
if min != nil && root.Left.Val <= *min {
return false
}
if max != nil && root.Left.Val >= *max {
return false
}
if !isValid(root.Left, min, &root.Val) {
return false
}
}
if root.Right != nil {
if root.Right.Val <= root.Val {
return false
}
if min != nil && root.Right.Val <= *min {
return false
}
if max != nil && root.Right.Val >= *max {
return false
}
if !isValid(root.Right, &root.Val, max) {
return false
}
}
return true
}
// @lc code=end
成绩:
- Your runtime beats 95.6 % of golang submissions
- Your memory usage beats 98.41 % of golang submissions (5.1 MB)
「真诚赞赏,手留余香」
真诚赞赏,手留余香
使用微信扫描二维码完成支付
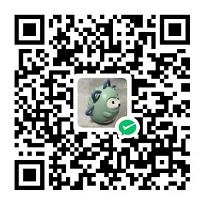